The Caesar Cipher, also known as a shift cypher, is one of the oldest and simplest forms of encrypting a message. It is a type of substitution cypher where each letter in the original message (which in cryptography is called the plaintext) is replaced with a letter corresponding to a certain number of letters shifted up or down in the alphabet.
For each letter of the alphabet, you would take its position in the alphabet, say 3 for the letter ‘C’, and shift it by the key number. If we had a key of +3, that ‘C’ would be shifted down to an ‘F’ – and that same process would be applied to every letter in the plaintext.
Mathematically,
Encryption = E n = (x + k) mod 26
Decryption = D n = ( x + k −1 ) mod 26
Where x is the input alphabet ASCII representation and k the key, k -1 is the additive inverse of k.
Code
#include "stdc++.h"
using namespace std;
string encrypt(string message, int key)
{
for (int i = 0; i < message.size(); i++)
{
message[i] = char(int(message[i] + key - 97) % 26 + 97);
}
return message;
}
string decrypt(string cipher, int key)
{
string message;
for (int i = 0; i < cipher.size(); i++)
{
int id = (cipher[i] - 'a' - key) % 26;
if (id >= 0)
message.push_back('a' + id);
else
message.push_back('z' + id);
}
return message;
}
int main()
{
cout << "Enter the text message to be encrypted: "
<< "\n";
string message;
cin >> message;
int key;
cout << "Enter the shift key: ";
cin >> key;
string cipher = encrypt(message, key);
cout << "The cipher is " << encrypt(message, key);
cout << "\nThe plaintext is " << decrypt(cipher, key);
return 0;
}
Output
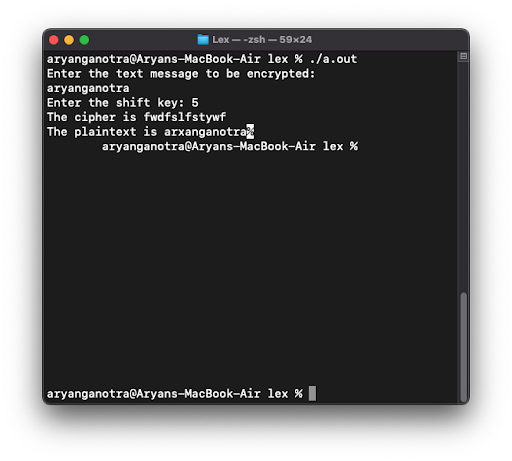