A monoalphabetic cipher scheme is one in which the characters of the plain text are mapped to the ciphertext in a one to one fashion, that is, every occurrence of a character in the plain text is mapped to the same ciphertext character.
For example, if ‘A’ is encrypted as ‘D’, for any number of occurrence in that plaintext, ‘A’ will always get encrypted to ‘D’.
Examples of monoalphabetic ciphers include the Caesar cipher, the atbash cipher etc.
An implementation of a monoalphabetic cipher is shown (Simple substitution cipher) :
Code
#include "stdc++.h"
// #include <bits/stdc++.h>
using namespace std;
unordered_map<char, char> hashMap;
unordered_map<char, char> reverseHashMap;
string encrypt(string plaintext) {
string ciphertext;
for (int i = 0; i < plaintext.size(); i++) {
ciphertext.push_back(hashMap[plaintext[i]]);
}
return ciphertext;
}
string decrypt(string cipher) {
string plaintext;
for (int i = 0; i < cipher.size(); i++) {
plaintext.push_back(reverseHashMap[cipher[i]]);
}
return plaintext;
}
void hashFn(string a, string b) {
hashMap.clear();
for (int i = 0; i < a.size(); i++) {
hashMap.insert(make_pair(a[i], b[i]));
reverseHashMap.insert(make_pair(b[i], a[i]));
}
}
int main() {
string alphabet = "abcdefghijklmnopqrstuvwxyz";
string substitution = "lzgwyorhvsbfmnpjtxqakciedu";
string txt;
cout << "enter the message\n";
cin >> txt;
cout << "\n";
hashFn(alphabet, substitution);
string cipher = encrypt(txt);
cout << "Encrypted Cipher Text: " << cipher << endl;
string plain = decrypt(cipher);
cout << "Decrypted Plain Text: " << plain << endl;
}
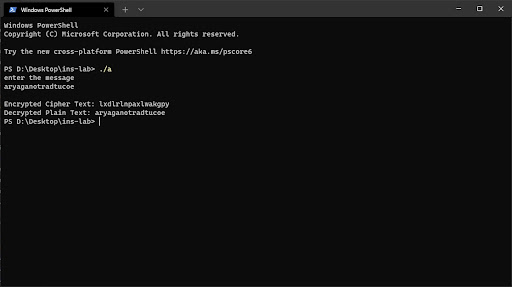