Playfair Cipher Decryption:
The decryption procedure is the same as encryption but the steps are applied in reverse order. For decryption the cipher is symmetric (move left along rows and up along columns). The receiver of the plain text has the same key and can create the same key-table that is used to decrypt the message.
Playfair cipher is an encryption algorithm to encrypt or encode a message. It is the same as a traditional cipher. The only difference is that it encrypts
a digraph (a pair of two letters) instead of a single letter.
The Playfair Cipher Encryption Algorithm: The Algorithm consists of 2 steps:
Generate the key Square(5×5):
- The key square is a 5×5 grid of alphabets that acts as the key for encrypting the plaintext. Each of the 25 alphabets must be unique and one letter of the alphabet (usually J) is omitted from the table (as the table can hold only 25 alphabets). If the plaintext contains J, then it is replaced by I.
- The initial alphabets in the key square are the unique alphabets of the key in the order in which they appear followed by the remaining letters of the alphabet in order.
Algorithm to encrypt the plaintext:
- The plain text is split into pairs of two letters (digraphs). If there is an odd number of letters, a Z is added to the last letter.
Code
#include "stdc++.h"
#include <stdlib.h>
#include <string.h>
#define SIZE 30
void toLowerCase(char plain[], int ps) {
int i;
for (i = 0; i < ps; i++) {
if (plain[i] > 64 && plain[i] < 91) plain[i] += 32;
}
}
int removeSpaces(char* plain, int ps) {
int i, count = 0;
for (i = 0; i < ps; i++)
if (plain[i] != ' ') plain[count++] = plain[i];
plain[count] = '\0';
return count;
}
void generateKeyTable(char key[], int ks, char keyT[5][5]) {
int i, j, k, flag = 0, *dicty;
dicty = (int*)calloc(26, sizeof(int));
for (i = 0; i < ks; i++) {
if (key[i] != 'j') dicty[key[i] - 97] = 2;
}
dicty['j' - 97] = 1;
i = 0;
j = 0;
for (k = 0; k < ks; k++) {
if (dicty[key[k] - 97] == 2) {
dicty[key[k] - 97] -= 1;
keyT[i][j] = key[k];
j++;
if (j == 5) {
i++;
j = 0;
}
}
}
for (k = 0; k < 26; k++) {
if (dicty[k] == 0) {
keyT[i][j] = (char)(k + 97);
j++;
if (j == 5) {
i++;
j = 0;
}
}
}
}
void search(char keyT[5][5], char a, char b, int arr[]) {
int i, j;
if (a == 'j')
a = 'i';
else if (b == 'j')
b = 'i';
for (i = 0; i < 5; i++) {
for (j = 0; j < 5; j++) {
if (keyT[i][j] == a) {
arr[0] = i;
arr[1] = j;
} else if (keyT[i][j] == b) {
arr[2] = i;
arr[3] = j;
}
}
}
}
int mod5(int a) { return (a % 5); }
int prepare(char str[], int ptrs) {
if (ptrs % 2 != 0) {
str[ptrs++] = 'z';
str[ptrs] = '\0';
}
return ptrs;
}
void encrypt(char str[], char keyT[5][5], int ps) {
int i, a[4];
for (i = 0; i < ps; i += 2) {
search(keyT, str[i], str[i + 1], a);
if (a[0] == a[2]) {
str[i] = keyT[a[0]][mod5(a[1] + 1)];
str[i + 1] = keyT[a[0]][mod5(a[3] + 1)];
} else if (a[1] == a[3]) {
str[i] = keyT[mod5(a[0] + 1)][a[1]];
str[i + 1] = keyT[mod5(a[2] + 1)][a[1]];
} else {
str[i] = keyT[a[0]][a[3]];
str[i + 1] = keyT[a[2]][a[1]];
}
}
}
void encryptByPlayfairCipher(char str[], char key[]) {
char ps, ks, keyT[5][5];
ks = strlen(key);
ks = removeSpaces(key, ks);
toLowerCase(key, ks);
ps = strlen(str);
toLowerCase(str, ps);
ps = removeSpaces(str, ps);
ps = prepare(str, ps);
generateKeyTable(key, ks, keyT);
encrypt(str, keyT, ps);
}
void decrypt(char str[], char keyT[5][5], int ps) {
int i, a[4];
for (i = 0; i < ps; i += 2) {
search(keyT, str[i], str[i + 1], a);
if (a[0] == a[2]) {
str[i] = keyT[a[0]][mod5(a[1] - 1)];
str[i + 1] = keyT[a[0]][mod5(a[3] - 1)];
} else if (a[1] == a[3]) {
str[i] = keyT[mod5(a[0] - 1)][a[1]];
str[i + 1] = keyT[mod5(a[2] - 1)][a[1]];
} else {
str[i] = keyT[a[0]][a[3]];
str[i + 1] = keyT[a[2]][a[1]];
}
}
}
void decryptByPlayfairCipher(char str[], char key[]) {
char ps, ks, keyT[5][5];
ks = strlen(key);
ks = removeSpaces(key, ks);
toLowerCase(key, ks);
ps = strlen(str);
toLowerCase(str, ps);
ps = removeSpaces(str, ps);
generateKeyTable(key, ks, keyT);
decrypt(str, keyT, ps);
}
int main() {
char str[SIZE], key[SIZE];
strcpy(key, "Ganotra");
printf("Key text: %s\n", key);
strcpy(str, "Aryan Ganotra DTU");
printf("Plain text: %s\n", str);
encryptByPlayfairCipher(str, key);
printf("Cipher text: %s\n", str);
printf("Decrypting the cipher...\n");
decryptByPlayfairCipher(str, key);
printf("Plain text after decryption is: Aryan Ganotra DTU");
return 0;
}
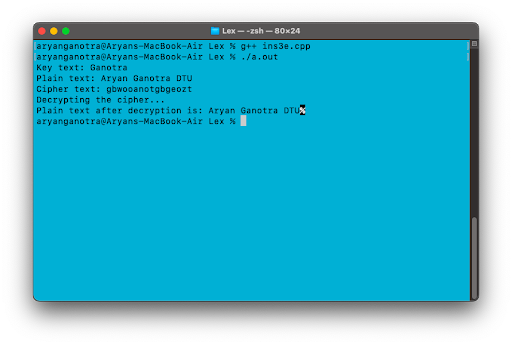